2022.11.13(更新日: 2022.12.02)
Vue.jsのウォッチャについて学んでみた
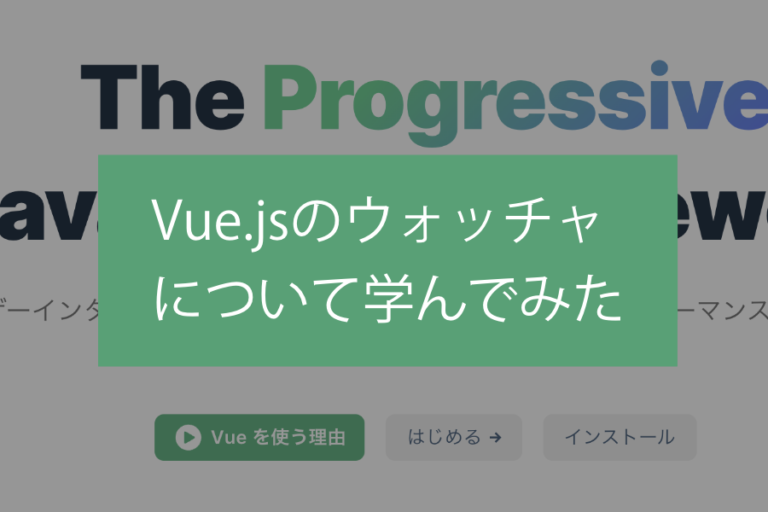
はじめに
vue.jsには、状態を監視する機能があります。
この記事では、ボタンを押すと3秒カウントして、
アラートを出すプログラムを作成していきます。
こちらの「chapter8」を参考にしました。
参考書のVue.jsのバージョンは2.5ですが、今回は3系の書き方に変更しました。
今回作成したプログラム
最初は「3秒」と「ボタン」が表示されています。
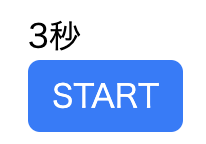
ボタンを押すとタイマーが動作します。
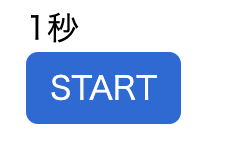
3秒経つとアラートが表示されます。
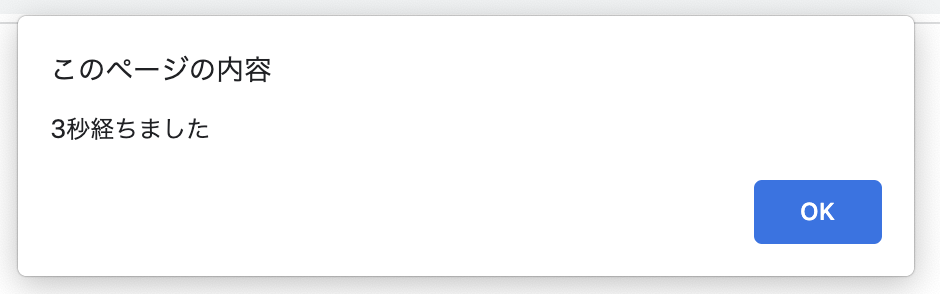
HTML・JSの記述
HTML・JSの記述で、必要な部分のみ抜粋して書いていきます。
こちらが、HTMLの記述です。
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
こちらが、JSの記述です。
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
次のセクションから、以下の流れでコードの解説をしていきます。
- 最初の画面になるまで
- ボタンを押してから
- 3秒たったあと
最初の画面になるまで
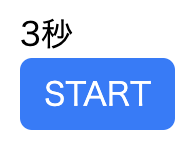
Vue.jsの読み込み
まずは、Vue.jsのコアファイルの読み込みが行われます。
HTML
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
DOMの読み込み
次に、idがappのDOMが読み込まれます。
HTML
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
3が表示される
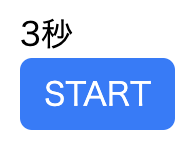
data関数が実行され、JSで定義した「restSecの3」が画面に表示されます。
timerObjは、ボタンを押した後の処理で使用します。
HTML
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
ボタンを押してから
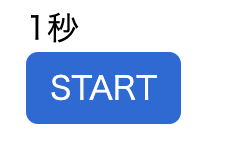
startTimer関数が発火する
ボタンを押すと、startTimer関数が発火します。
HTML
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
1秒ごとに秒数が減っていく
startTimer関数では、1秒(1,000ミリ秒)ごとに、restSecの3という数字が減っていくという処理を行なっています。
HTML
<script src="https://unpkg.com/vue@3/dist/vue.global.js"></script>
</head>
<body>
<div id="app">
<p>{{ restSec }}秒<br>
<button v-on:click="startTimer">START</button>
</div>
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
3秒たったあと
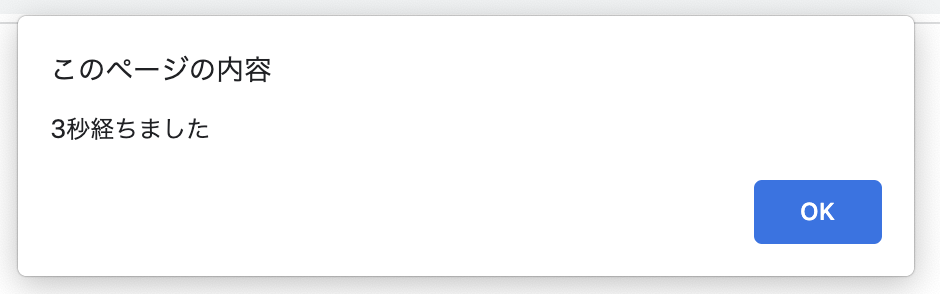
ウォッチャー
そもそも、ウォッチプロパティーで、restSecを監視していました。
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
3秒たった時の処理
「resrSec」が0以下になった時に「3秒経ちました」というアラートを表示させ、1秒ごとのカウントダウンを停止しています。
JS
Vue.createApp({
data() {
return {
restSec: 3,
timerObj: null,
}
},
methods: {
startTimer: function () {
this.restSec = 3;
this.timerObj = setInterval(() => { this.restSec-- }, 1000)
}
},
watch: {
restSec: function () {
if (this.restSec <= 0) {
alert("3秒経ちました");
clearInterval(this.timerObj);
}
}
}
}).mount("#app");
投稿ID : 6090
コメントを残す